We Guarantee the Best and Free technical solutions
Some Usefull link in this Application will redirect your mind to a new techical world
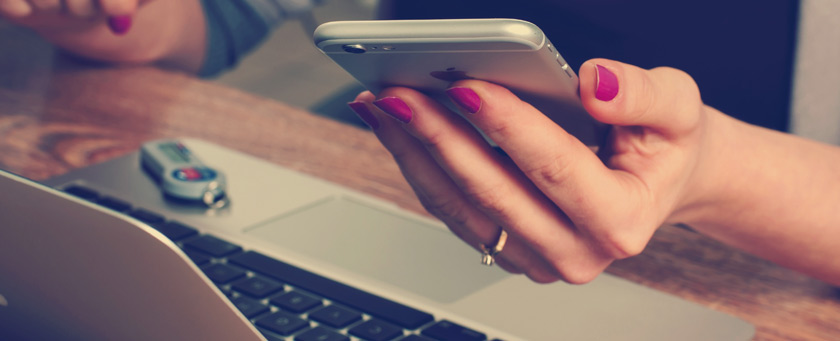
File upload in Laravel
Here i m giving you a sample code for file upload in laravel.This code contains only file upload there is no validation added here.Files are storing into the public/uploads folder.uploaded file details are stored in the database.
Step1:database Setup and table creation
added the database details in the .env file
DB_HOST=localhost
DB_DATABASE=laravel-practice
DB_USERNAME=root
DB_PASSWORD=
you can check the db configuration in the config/database.php for more information related to database connectivity
create database table using the below sql statement.
CREATE TABLE IF NOT EXISTS `tbl_file_upload` (
`id` int(11) NOT NULL,
`orginal_fie_name` varchar(250) NOT NULL,
`saved_file_name` varchar(250) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
ALTER TABLE `tbl_file_upload`
ADD PRIMARY KEY (`id`);
ALTER TABLE `tbl_file_upload`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
Step2:Setting the upload path through constants.php file inside the config folder.create a file called constants.php, and add the below code
return [
'FILE_PATHS' => [
'UPLOAD_FILE_PATH' => public_path(). DIRECTORY_SEPARATOR . "uploads". DIRECTORY_SEPARATOR . "myfiles"]
];
Step3:Setting the route files.Inside the routes.php file add the below lines of code.
your url will be looks like projectfolder/public/fileupload, this will call the index function inside the controller from the index function it will show the view page for file upload
second function in the routes.php do the upload functionalities and db insertion.
Route::get('fileupload/', 'FileUploadController@index');
Route::post('uploadfile/', 'FileUploadController@upLoadFile');
Controller file be looks like as below
namespace App\Http\Controllers;
use Session;
use App\Http\Requests;
use App\Http\Controllers\Controller;
use App\Students;
use Illuminate\Http\Request;
use Config;
use App\Models\FileUpload;
class FileUploadController extends Controller {
public function index()
{
$data['title']='File Upload Using Laravel';
return view('fileupload.fileupload',$data);
}
public function uploadfile(Request $request)
{
if ($request->hasFile('file_name')) {
if ($request->file('file_name')->isValid())
{
$file = $request->file('file_name');
$originalName = $file->getClientOriginalName();
$rawName = pathinfo($originalName, PATHINFO_FILENAME);
$extension = $file->getClientOriginalExtension();
$destinationPath = Config::get('constants.FILE_PATHS.UPLOAD_FILE_PATH');
$getDate = date("Ymd");
$getTime = time();
$neFileName = $rawName . $getDate . '_' . $getTime . mt_rand(5, 100). "." . $extension;
$file->move($destinationPath, $neFileName);
$data=array('orginal_fie_name'=>$originalName,'saved_file_name'=>$neFileName);
FileUpload::insert($data);
}
}
return redirect('fileupload');
}
}
In the controller we are creating a new file using timestamp.Timestamp is appending to the origanal uploaded file name to avoid duplication if upload same file multiple times.
Step5:Model File Creation create model file using php artisan command or else you can directly create model file inside the Models folder.here Fileupload.php model file has been created
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
use App\Models\FileUpload;
/**
* Class MilestoneManagement
*/
class FileUpload extends Model {
protected $table = 'tbl_file_upload';
protected $primaryKey = 'id';
public $timestamps = false;
protected $fillable = [
'orginal_fie_name',
'saved_file_name'
];
protected $guarded = [];
}
Step6:Finally you have to create the view file.This blade file stored inside the resources/view/fileupload folder.create the blade fileupload.blade.php
File upload using Laravel
{!! Form::open(array('url' => url('/uploadfile'), 'class'=>'form-horizontal', 'id'=>'fileUploadeForm','files' => true)) !!}
{!! Form::close() !!}