We Guarantee the Best and Free technical solutions
Some Usefull link in this Application will redirect your mind to a new techical world
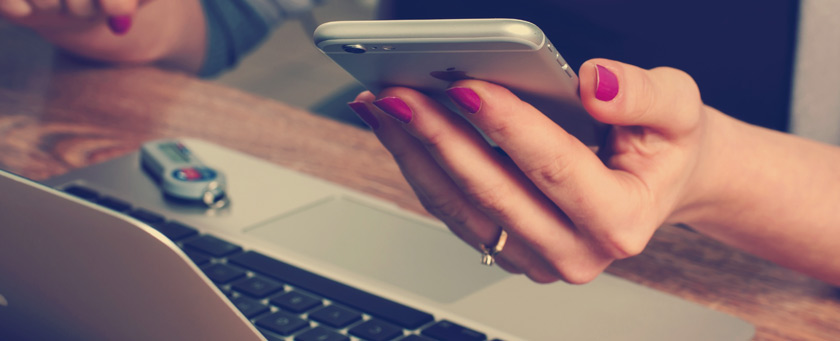
Sorting elements in a grid using mouse--Sorted grid elements display using jquery and codeigniter
Complete script and demo of grid element reordering is explained here. Codeigniter and jquery is used to do this functionality, A Grid of employee list with First_name,Last_name,Email,Country,State,City,Age are displayed based on how the ordering was done. Below are the controler and models used to create the jquery sort element using codeigniter.
Step1:Create the database using the following Mysql query.
CREATE TABLE IF NOT EXISTS `list_order` (
`id` int(10) NOT NULL AUTO_INCREMENT,
`login_id` int(10) NOT NULL,
`field_name` varchar(50) NOT NULL,
`order` int(2) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=7 ;
INSERT INTO `list_order` (`id`, `login_id`, `field_name`, `order`) VALUES
(1, 1, 'first_name', 4),
(2, 1, 'last_name', 3),
(3, 1, 'email', 5),
(4, 1, 'state', 1),
(5, 1, 'city', 2),
(6, 1, 'age', 6);
CREATE TABLE IF NOT EXISTS `tbl_employee` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`first_name` varchar(50) NOT NULL,
`last_name` varchar(50) NOT NULL,
`email` varchar(50) NOT NULL,
`country` varchar(50) NOT NULL,
`state` varchar(50) NOT NULL,
`city` varchar(50) NOT NULL,
`age` int(2) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=4 ;
--
-- Dumping data for table `tbl_employee`
--
INSERT INTO `tbl_employee` (`id`, `first_name`, `last_name`, `email`, `country`, `state`, `city`, `age`) VALUES
(1, 'vasanthan', 'pv', 'vasanthanpv@gmail.com', 'india', 'kerala', 'mangalore', 33),
(2, 'Martin', 'jacob', 'mrt@myemail.com', 'india', 'karnataka', 'mangalore', 30),
(3, 'Sathyan', 'p', 'sthy@m', 'india', 'kerala', 'kannur', 28);
Below are the controllers and models files used in the sorting application Controller: grid_element_sorting_using_jquery_php_mysql.php
if ( ! defined('BASEPATH')) exit('No direct script access allowed');
class Grid_element_sorting_using_jquery_php_mysql extends CI_Controller {
function __construct(){
parent::__construct();
$this->load->model('list_order_model', '', TRUE);
$this->load->model('list_employee_model', '', TRUE);
}
public function grid_sort_using_jquery_php()
{
$this->load->view('grid_element_sorting_using_jquery_php_mysql/grid_element_sorting_using_jquery_php_mysql');
}
public function demo_grid_element_sorting_using_jquery_php_mysql()
{
$login_id='1';// here only single student so its hardcoded
$data['list_order']=$this->list_order_model->get_list_order($login_id);
$this->load->view('grid_element_sorting_using_jquery_php_mysql/demo_grid_element_sorting_using_jquery_php_mysql',$data);
}
public function update_list_order()
{
$list_order_id=$this->input->post('list_order_id', TRUE);
$explode_data=explode(",",$list_order_id);
$i=1;
foreach($explode_data as $data_val)
{
$data = array('order' => $i);
$this->list_order_model->update_order($data,$data_val);
$i++;
}
$login_id=1;
$data['list_of_employee']=$this->list_employee_model->employee_list($login_id);
$data['list_order']=$this->list_order_model->get_list_order($login_id);
$this->load->view('grid_element_sorting_using_jquery_php_mysql/load_employee_list',$data);
}
public function initial_load_data()
{
$login_id=1;
$data['list_of_employee']=$this->list_employee_model->employee_list($login_id);
$data['list_order']=$this->list_order_model->get_list_order($login_id);
$this->load->view('grid_element_sorting_using_jquery_php_mysql/load_employee_list',$data);
}
}
Model:list_employee_model
class List_employee_model extends CI_Model {
function __construct() {
// Call the Model constructor
parent::__construct();
}
function employee_list()
{
$this->db->select('*');
$query_result= $this->db->get('tbl_employee');
$data['num_rows']=$query_result->num_rows();
if($query_result->num_rows()>0)
{
$data['result']=$query_result->result_array();
}
return $data;
}
}
Model: list_order_model
class List_order_model extends CI_Model {
function __construct() {
// Call the Model constructor
parent::__construct();
}
function get_list_order($login_id)
{
$this->db->select('*');
$this->db->where('list_order.login_id',$login_id);
$this->db->order_by("order", "asc");
$query_result= $this->db->get('list_order');
$data['num_rows']=$query_result->num_rows();
if($query_result->num_rows()>0)
{
$data['result']=$query_result->result_array();
}
return $data;
}
function update_order($data,$data_val)
{
$this->db->where('id', $data_val);
$this->db->update('list_order', $data);
}
}
create a view file in the folder "grid_element_sorting_using_jquery_php_mysql". View:demo_grid_element_sorting_using_jquery_php_mysql.php
Jquery grid examples and demo,dynamic column sort in grid using jquery
if($list_order['num_rows']>0)
{
foreach($list_order['result'] as $list_order_result)
{
- echo $list_order_result['field_name'];
}
}
Click to see the Demo